NodeJS Save Form Data MySQL
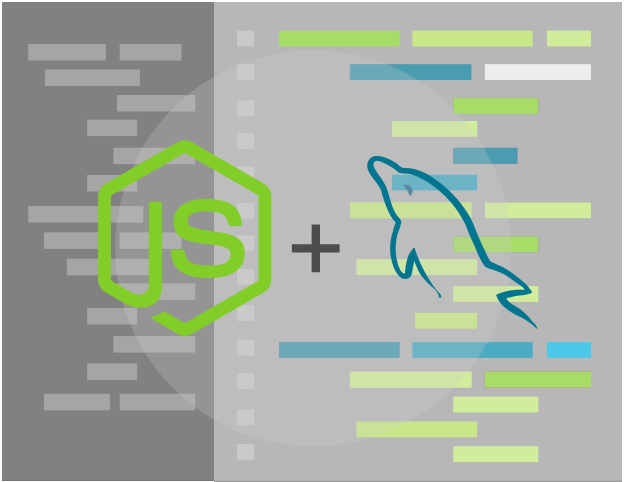
NodeJS is a popular open-source, cross-platform JavaScript runtime environment that is used for server-side scripting. It provides a fast and efficient way of building scalable network applications, and it is especially useful for real-time data-intensive applications.
One of the most common use cases for NodeJS is building web forms, which allow users to submit data to a server for processing. In this article, we will be discussing how to save form data in a MySQL database table using NodeJS.
NodeJS is a great choice for web developers who want to build fast, efficient, and scalable web applications. With its growing popularity and robust feature set, it’s no wonder that many developers are turning to NodeJS for their web development needs.
When building web forms, it is often necessary to save the form data in a database for later use. In this article, we will be discussing how to save form data in a MySQL database table using NodeJS.
Saving Form Data in a MySQL Database Table with NodeJS:
To save form data in a MySQL database table using NodeJS, you will need to follow these steps:
- Set up a MySQL database and create a table to store the form data.
- Install the MySQL module for NodeJS.
- Connect to the MySQL database using the NodeJS MySQL module.
- Write the code to insert the form data into the MySQL database table.
Step 1: Setting up a MySQL Database and Table
The first step in saving form data in a MySQL database table using NodeJS is to set up a MySQL database and create a table to store the form data. To do this, you can use a web-based MySQL management tool such as phpMyAdmin or use the MySQL command-line interface.
Here’s an example of how you can create a table to store form data in a MySQL database:
CREATE TABLE form_data (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255),
email VARCHAR(255),
message TEXT
);
Step 2: Installing the MySQL Module for NodeJS
The next step is to install the MySQL module for NodeJS. The MySQL module for NodeJS provides a simple way to connect to and interact with a MySQL database. To install the MySQL module, you can use the following command:
npm install mysql
Step 3: Connecting to the MySQL Database
Once you have installed the MySQL module, you can use it to connect to the MySQL database. To connect to the database, you will need to provide the following information:
- The database host
- The database name
- The database user name
- The database password
Here’s an example of how you can connect to a MySQL database using NodeJS:
var mysql = require('mysql');
var con = mysql.createConnection({
host: 'localhost',
user: 'yourusername',
password: 'yourpassword',
database: 'yourdatabasename',
});
con.connect(function (err) {
if (err) throw err;
console.log('Connected to the MySQL database!');
});
Step 4: Writing the Code to Insert Form Data into the MySQL Database Table
Once you have successfully connected to the MySQL database, you can use the following code to insert form data into the database table:
app.post('/form_submit', function (req, res) {
var sql = 'INSERT INTO form_data (name, email, message) VALUES (?, ?, ?)';
var values = [req.body.name, req.body.email, req.body.message];
con.query(sql, values, function (err, result) {
if (err) throw err;
console.log('Form data inserted into the database');
});
});
In this code, we are using the app.post
method to handle a form submit event.
The req.body
object contains the form data that was submitted, and we are using the con.query
method to insert this data into the MySQL database table.
Conclusion:
NodeJS is a powerful platform for building web applications, and it is particularly well-suited for real-time data-intensive applications. By following the steps outlined in this article, you can easily save form data in a MySQL database table using NodeJS. Whether you are a beginner or an experienced web developer, this is a simple and straightforward process that can be completed in just a few minutes.