How Storing Data in a Pivot Table in Laravel
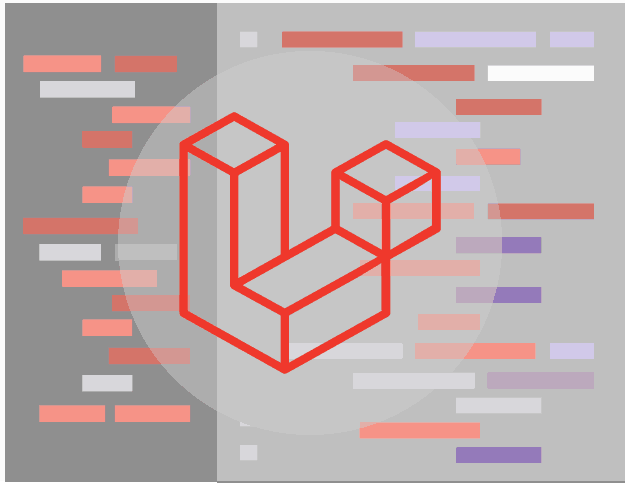
How Storing Data in a Pivot Table in Laravel
Laravel is a popular PHP framework used for web development, and it provides a simple and elegant way to store data in a pivot table.
A pivot table is a special type of table in a relational database that allows you to store many-to-many relationships between two models. In Laravel, you can use the Eloquent ORM to store data in a pivot table and retrieve it as needed. To store data in a pivot table in Laravel, you need to follow these steps:
Create two models that you want to relate to each other using the Artisan command line tool:
php artisan make:model User php artisan make:model Role
Create a pivot table in your database using the Schema builder:
Schema::create('role_user', function (Blueprint $table) { $table->unsignedBigInteger('user_id'); $table->unsignedBigInteger('role_id'); $table->timestamps(); });
Define the many-to-many relationship between the two models using the Eloquent ORM:
User.php
public function roles() { return $this->belongsToMany(Role::class); }
Role.php
public function users() { return $this->belongsToMany(User::class); }
Store data in the pivot table using the attach or sync methods in the controller:
// Attach $user->roles()->attach($roleId); // Sync $user->roles()->sync([$roleId1, $roleId2, ...]);
Following these steps, you can easily store data in a pivot table in Laravel and retrieve it as needed. This allows you to manage relationships between multiple models and store data in a simple and organized way. Laravel provides an easy-to-use interface for working with pivot tables and other relational data, making it a popular choice for web developers.